The Smart Helmet project enhances motorcycle safety by integrating advanced sensor technology with an Arduino Uno microcontroller. It addresses critical aspects of rider safety through real-time monitoring and activation mechanisms.
Components Required:
- Arduino Uno
- Pulse sensor module
- Touch sensor
- LEDs (Red and white)
- Resistors
- Relay module (to represent a bike starting circuit)
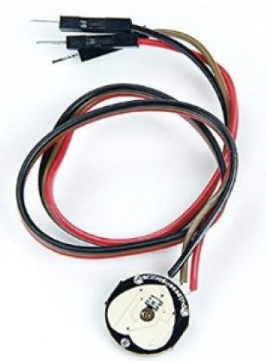
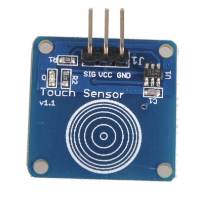
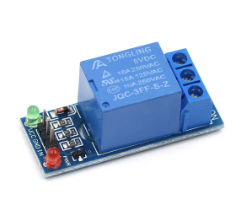
Circuit Connections:
- Pulse Sensor: Connects to Arduino analog pin A3 for pulse rate detection.
- Touch Sensor: Connected to Arduino digital pin 2 with internal pull-up resistor.
- LEDs:
- Red LED (warning) connected to pin 5.
- White LED (bike start indication) connected to pin 6.
- Relay Module: Connects to Arduino digital pin 3.
The circuit diagram is given below:
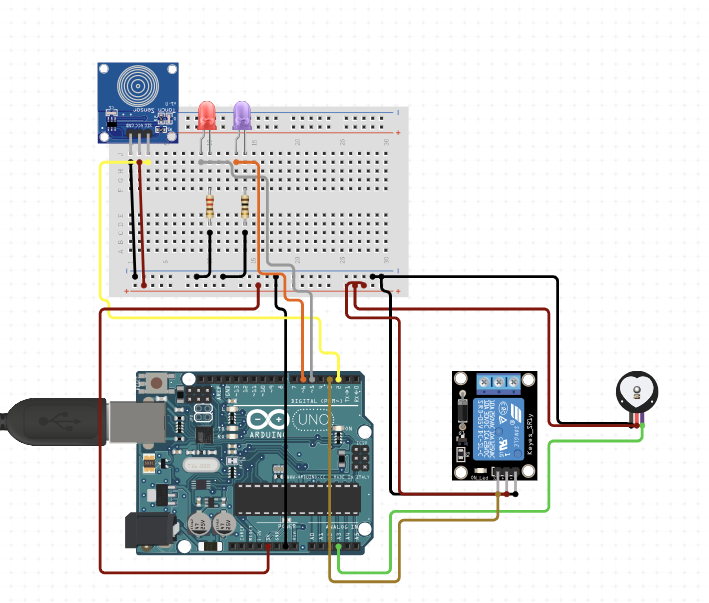
Working:
The pulse sensor, positioned on the helmet’s belt, continuously monitors the wearer’s pulse rate. When the helmet is securely fastened, indicating safety compliance, the pulse sensor detects and sends pulse data to the Arduino Uno.
Simultaneously, the touch sensor, integrated into the helmet’s surface, detects taps. To start the motorcycle, the rider must tap the touch sensor three times consecutively. This action triggers the Arduino Uno to initiate the bike’s ignition sequence, ensuring deliberate activation for enhanced safety.
The code is given below:
// Define pin numbers for components
const int pulseSensorPin = A3; // Pulse sensor connected to analog pin A0
const int touchSensorPin = 2; // Touch sensor connected to digital pin 2
const int startLedPin = 6; // LED for indicating bike start status
//const int pulseDetectedLedPin = 8; // LED for indicating pulse detected status
const int warningLedPin = 5; // Red LED for warning when belt is not buckled
const int BikeEngine = 3; //Use a relay module to represent the bike engine
// Variables
int pulseThreshold = 50; // Adjust this threshold as per your pulse sensor readings
int pulseValue = 0; // Variable to store pulse sensor readings
bool pulseDetected = false; // Flag to indicate if pulse is detected
bool helmetBuckled = false; // Flag to indicate if helmet is buckled
bool bikeStarted = false; // Flag to indicate if bike is started by touching sensor
void setup() {
Serial.begin(9600); // Initialize serial communication for debugging
pinMode(touchSensorPin, INPUT_PULLUP); // Set touch sensor pin as input with internal pull-up resistor
pinMode(startLedPin, OUTPUT); // Set start LED pin as output
//pinMode(pulseDetectedLedPin, OUTPUT); // Set pulse detected LED pin as output
pinMode(warningLedPin, OUTPUT); // Set warning LED pin as output
pinMode(BikeEngine, OUTPUT);
// Additional setup code for sensors, if required
}
void loop() {
// Read pulse sensor value
pulseValue = analogRead(pulseSensorPin);
// Check if helmet is buckled
helmetBuckled = digitalRead(touchSensorPin) == LOW; // Touch sensor is active low
// Check if pulse is detected
if (pulseValue > pulseThreshold) {
pulseDetected = true;
} else {
pulseDetected = false;
}
// Blink red warning LED if helmet is not buckled
if (!helmetBuckled) {
digitalWrite(warningLedPin, HIGH); // Turn on warning LED
delay(500); // Blink delay
digitalWrite(warningLedPin, LOW); // Turn off warning LED
delay(500); // Blink delay
}
// Logic for controlling bike start based on conditions
if (helmetBuckled && !bikeStarted && pulseDetected) {
digitalWrite(BikeEngine, HIGH); // Turn on the relay to show bike has started
digitalWrite(startLedPin, HIGH); // Turn on start LED
bikeStarted = true; // Set bike started flag
} else if (!helmetBuckled || !pulseDetected) {
digitalWrite(BikeEngine, LOW); // Ensure the bike is not started if pulse si not detected
digitalWrite(startLedPin, LOW); // Turn off start LED
bikeStarted = false; // Reset bike started flag
}
// Visual indication of pulse detection
/* if (pulseDetected) {
digitalWrite(pulseDetectedLedPin, HIGH); // Turn on pulse detected LED
} else {
digitalWrite(pulseDetectedLedPin, LOW); // Turn off pulse detected LED
}*/
// Check for touch sensor input to start the bike
checkTouchSensor();
// Serial monitor output for debugging (optional)
Serial.print("Pulse Value: ");
Serial.println(pulseValue);
Serial.print("Helmet Buckled: ");
Serial.println(helmetBuckled);
delay(100); // Delay for stability, adjust as necessary
}
// Function to check touch sensor input for bike start
void checkTouchSensor() {
static int touchCount = 0; // Static variable to count touches
if (digitalRead(touchSensorPin) == LOW) {
// Touch sensor is active low
touchCount++;
// Delay to debounce and prevent multiple counts for a single touch
delay(50);
}
if (touchCount >= 3) {
// If touched 3 times consecutively
bikeStarted = false; // Reset bike started flag to require pulse detection again
touchCount = 0; // Reset touch count
// Additional actions to start the bike here
// For example, activate a relay or send a start signal
digitalWrite(startLedPin, HIGH); // Turn on start LED
// Delay to stabilize the start action
delay(1000);
// Ensure bike started flag is set after successful start
bikeStarted = true;
}
}
Features of the Project:
- Safety Enhancement: Ensures the helmet is correctly fastened before allowing bike start.
- User Interaction: Requires intentional taps on the helmet for bike activation.
- Real-time Monitoring: Continuously monitors pulse rate for rider safety
Application:
Motorcycle Safety Systems:
- Enhances overall motorcycle safety by ensuring that the rider is wearing the helmet properly before starting the bike.
- Reduces the risk of head injuries by promoting consistent helmet use.
Commuter and Delivery Services:
- Ideal for companies with delivery personnel, ensuring that their employees adhere to safety protocols and reduce the risk of accidents.
Personal Safety for Riders:
- Provides individual riders with an additional safety layer, particularly useful for long-distance and high-speed travel.
- Alerts riders immediately if the helmet is not properly secured, helping to prevent accidents due to negligence.