Overview
Create a simple and effective Distance Measuring Device using an ultrasonic sensor, Arduino, and buzzer. This project is perfect for detecting objects and measuring distances with an audible alert when objects are too close.
Project Description: Distance Measuring Device with Buzzer Alert and LCD Display
Overview
Create a simple and effective Distance Measuring Device using an ultrasonic sensor, Arduino, buzzer, and an I2C LCD. This project is perfect for detecting objects and measuring distances with a visual display and an audible alert when objects are too close.
Key Features
- Accurate Distance Measurement: Measures distance using an ultrasonic sensor.
- Audible Alerts: Buzzer sounds when objects are too close.
- LCD Display: Shows real-time distance measurements.
- Easy to Use: Simple setup and operation.
Components Used
- Arduino: The brain of the project.
- HC-SR04 Ultrasonic Sensor: Measures distance to objects.
- Buzzer: Provides an audible alert.
- I2C LCD: Displays distance measurements.
- Jumper Wires: Connects components.
- Breadboard (optional): For easy wiring.
How It Works
- Ultrasonic Sensor: Sends out a sound wave and measures the time it takes for the echo to return.
- Distance Calculation: Arduino calculates the distance based on the echo time.
- LCD Display: Shows the distance on the screen.
- Buzzer Alert: If the object is closer than the set distance, the buzzer sounds.
Connections
- HC-SR04 Sensor:
- VCC to 5V on Arduino
- GND to GND on Arduino
- Trig to Digital Pin 3 on Arduino
- Echo to Digital Pin 4 on Arduino
- Buzzer:
- Positive to Digital Pin 2 on Arduino
- Negative to GND on Arduino
- I2C LCD:
- SDA to A4 on Arduino
- SCL to A5 on Arduino
- VCC to 5V on Arduino
- GND to GND on Arduino
Benefits
- Improves Safety: Alerts you when objects are too close.
- Educational: Great for learning about electronics and programming.
- Customizable: Easily adjust the alert distance.
Applications
- Parking Sensors: Helps in detecting obstacles while parking.
- Robotics: Use in robots to avoid obstacles.
- Home Security: Detects intruders or objects moving too close.
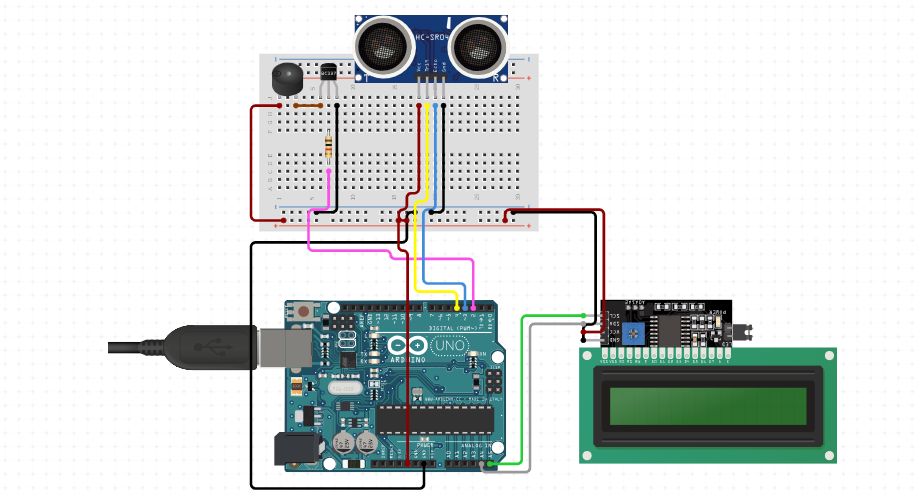
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Initialize the LCD library with the I2C address and LCD size (16 columns and 2 rows)
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Define pins for HC-SR04
const int trigPin = 3;
const int echoPin = 4;
// Define pin for Buzzer
const int buzzerPin = 2;
// Define threshold distance in centimeters
const int thresholdDistance = 20;
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Initialize pins
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(buzzerPin, OUTPUT);
// Ensure the buzzer is off at startup
digitalWrite(buzzerPin, LOW);
// Initialize the LCD
lcd.begin();
lcd.backlight();
lcd.setCursor(0, 0);
lcd.print("Distance: ");
}
void loop() {
// Send a 10us pulse to trigger pin to start measurement
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Read the echo pin, returns the sound wave travel time in microseconds
long duration = pulseIn(echoPin, HIGH);
// Calculate the distance in centimeters
long distance = duration * 0.034 / 2;
// Print the distance to the serial monitor
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
// Display the distance on the LCD
lcd.setCursor(10, 0);
lcd.print(" "); // Clear previous value
lcd.setCursor(10, 0);
lcd.print(distance);
lcd.print(" cm");
// Check if the distance is less than the threshold
if (distance <= thresholdDistance) {
// If object is too close, turn on the buzzer
digitalWrite(buzzerPin, HIGH);
} else {
// If object is far enough, turn off the buzzer
digitalWrite(buzzerPin, LOW);
}
// Wait for 500 milliseconds before the next measurement
delay(500);
}